问题描述
给定一个二叉树 root
,返回其最大深度。
二叉树的 最大深度 是指从根节点到最远叶子节点的最长路径上的节点数。
示例 1:
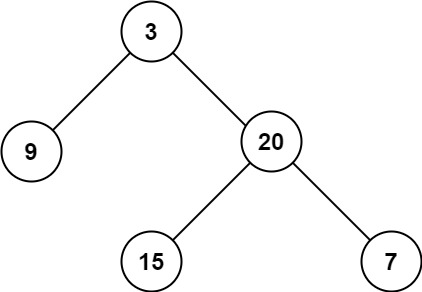
输入:root = [3,9,20,null,null,15,7]
输出:3
示例 2:
输入:root = [1,null,2]
输出:2
提示:
- 树中节点的数量在
[0, 104]
区间内。
-100 <= Node.val <= 100
核心思路
本题可以使用前序(中左右),也可以使用后序遍历(左右中),使用前序求的就是深度,使用后序求的是高度。
- 二叉树节点的深度:指从根节点到该节点的最长简单路径边的条数或者节点数(取决于深度从0开始还是从1开始)
- 二叉树节点的高度:指从该节点到叶子节点的最长简单路径边的条数或者节点数(取决于高度从0开始还是从1开始)
而根节点的高度就是二叉树的最大深度,所以本题中我们可以通过后序求的根节点高度来求得二叉树最大深度。
实现要点
本题遍历只能是“后序遍历”,因为我们要通过递归函数的返回值来判断两个子树的内侧节点和外侧节点是否相等。
不过,准确的来说,一棵树的遍历顺序是左右中,另一棵树的遍历顺序是右左中。不是严格上的后序遍历,但可以理解算是后序遍历。
同时,后序也可以理解为是一种回溯。
递归
后序
考虑递归三部曲。
1 2 3 4 5 6 7 8 9 10 11 12 13
| class solution { public: int getdepth(TreeNode* node) { if (node == NULL) return 0; int leftdepth = getdepth(node->left); int rightdepth = getdepth(node->right); int depth = 1 + max(leftdepth, rightdepth); return depth; } int maxDepth(TreeNode* root) { return getdepth(root); } };
|
前序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class solution { public: int result; void getdepth(TreeNode* node, int depth) { result = depth > result ? depth : result;
if (node->left == NULL && node->right == NULL) return ;
if (node->left) { depth++; getdepth(node->left, depth); depth--; } if (node->right) { depth++; getdepth(node->right, depth); depth--; } return ; } int maxDepth(TreeNode* root) { result = 0; if (root == NULL) return result; getdepth(root, 1); return result; } };
|
迭代
使用迭代法的话,使用层序遍历是最为合适的,因为最大的深度就是二叉树的层数,和层序遍历的方式极其吻合。
在二叉树中,一层一层的来遍历二叉树,记录一下遍历的层数就是二叉树的深度。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| class solution { public: int maxDepth(TreeNode* root) { if (root == NULL) return 0; int depth = 0; queue<TreeNode*> que; que.push(root); while(!que.empty()) { int size = que.size(); depth++; for (int i = 0; i < size; i++) { TreeNode* node = que.front(); que.pop(); if (node->left) que.push(node->left); if (node->right) que.push(node->right); } } return depth; } };
|