问题描述
给定一个二叉树,找出其最小深度。
最小深度是从根节点到最近叶子节点的最短路径上的节点数量。
说明:叶子节点是指没有子节点的节点。
示例 1:
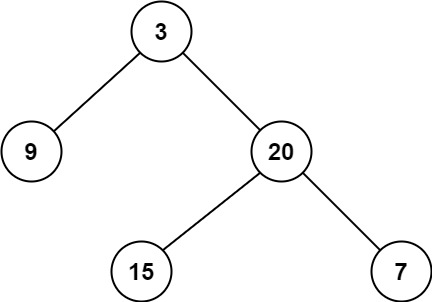
输入:root = [3,9,20,null,null,15,7]
输出:2
示例 2:
输入:root = [2,null,3,null,4,null,5,null,6]
输出:5
提示:
- 树中节点数的范围在
[0, 105]
内
-1000 <= Node.val <= 1000
核心思路
和求最大深度类似,本题用前序遍历和后序遍历都可以,前序求的是深度,后序求的是高度。
- 二叉树节点的深度:指从根节点到该节点的最长简单路径边的条数或者节点数(取决于深度从0开始还是从1开始)
- 二叉树节点的高度:指从该节点到叶子节点的最长简单路径边的条数后者节点数(取决于高度从0开始还是从1开始)
那么使用后序遍历,其实求的是根节点到叶子节点的最小距离,就是求高度的过程,不过这个最小距离也同样是最小深度。
实现要点
最小深度是从根节点到最近叶子节点的最短路径上的节点数量。求二叉树的最小深度和最大深度的差别主要在于处理左右孩子不为空的逻辑。
递归
后序
考虑递归三部曲。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class Solution { public: int getDepth(TreeNode* node) { if (node == NULL) return 0; int leftDepth = getDepth(node->left); int rightDepth = getDepth(node->right); if (node->left == NULL && node->right != NULL) { return 1 + rightDepth; } if (node->left != NULL && node->right == NULL) { return 1 + leftDepth; } int result = 1 + min(leftDepth, rightDepth); return result; }
int minDepth(TreeNode* root) { return getDepth(root); } };
|
前序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
| class Solution { private: int result; void getdepth(TreeNode* node, int depth) { if (node == nullptr) { return; } if (node -> left == nullptr && node->right == nullptr) { result = min(result, depth); } if (node->left) { getdepth(node->left, depth + 1); } if (node->right) { getdepth(node->right, depth + 1); } return ; }
public: int minDepth(TreeNode* root) { if (root == nullptr) { return 0; } result = INT_MAX; getdepth(root, 1); return result; } };
|
迭代
适合层序遍历。需要注意的是,只有当左右孩子都为空的时候,才说明遍历到最低点了。如果其中一个孩子不为空则不是最低点。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| class Solution { public:
int minDepth(TreeNode* root) { if (root == NULL) return 0; int depth = 0; queue<TreeNode*> que; que.push(root); while(!que.empty()) { int size = que.size(); depth++; for (int i = 0; i < size; i++) { TreeNode* node = que.front(); que.pop(); if (node->left) que.push(node->left); if (node->right) que.push(node->right); if (!node->left && !node->right) { return depth; } } } return depth; } };
|